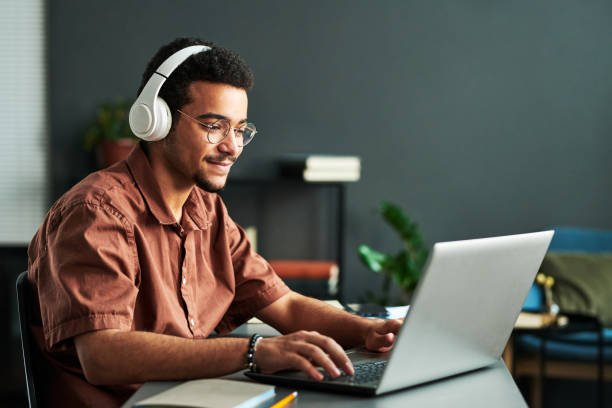
MATLAB, short for Matrix Laboratory, is a programming environment widely used for numerical computing, data analysis, and algorithm development. While its applications are extensive, students and professionals often find themselves grappling with the complexity of MATLAB assignments. This blog post explores a real-world case study to illustrate strategies for tackling a challenging MATLAB project step-by-step.
Understanding the Assignment Requirements
Decoding the Problem Statement
The first step in any MATLAB assignment is to thoroughly understand the problem statement. For instance, consider an assignment that involves:
-
Simulating a dynamic system (e.g., a pendulum or electrical circuit).
-
Analyzing data to identify trends or anomalies.
-
Creating a GUI for user interaction.
In this case study, the assignment requires simulating a predator-prey model using differential equations and visualizing the results.
Identifying Key Deliverables
Key deliverables for this assignment include:
-
Writing MATLAB code to model predator-prey dynamics.
-
Plotting population changes over time.
-
Validating the model using predefined parameters.
-
Including comments for code clarity.
A clear understanding of these deliverables is crucial for planning and execution.
Planning and Organizing the Assignment
Breaking Down the Problem
Complex assignments can be daunting. Breaking them into smaller tasks makes the process manageable:
-
Research and Review: Familiarize yourself with predator-prey dynamics and relevant mathematical models, such as the Lotka-Volterra equations.
-
Algorithm Design: Outline the logic and steps required for simulation.
-
Coding: Translate the algorithm into MATLAB code.
-
Debugging and Testing: Verify the correctness of your implementation.
-
Visualization: Create graphs or other visual representations of the results.
Setting a Timeline
Divide the tasks across a timeline to ensure steady progress:
-
Day 1: Research and outline the problem.
-
Day 2-3: Design and write the initial code.
-
Day 4: Test and debug.
-
Day 5: Finalize visualization and add comments.
-
Day 6: Review and submit.
Implementing the Solution
Step 1: Setting Up MATLAB
Before diving into coding, set up the MATLAB environment:
-
Install necessary toolboxes (e.g., Simulink or Symbolic Math Toolbox).
-
Create a new script or function file for the project.
Step 2: Writing the Code
Below is an example of MATLAB code to simulate the Lotka-Volterra model:
% Lotka-Volterra Predator-Prey Model % Define parameters alpha = 0.1; % Prey birth rate beta = 0.02; % Predation rate gamma = 0.1; % Predator death rate delta = 0.01; % Predator reproduction rate
% Initial populations prey = 40; predator = 9;
% Time span tspan = [0 200];
% Define differential equations function dydt = lotka_volterra(t, y) prey = y(1); predator = y(2); dydt = [ alpha * prey - beta * prey * predator; delta * prey * predator - gamma * predator ]; end
% Solve ODEs [t, y] = ode45(@lotka_volterra, tspan, [prey, predator]);
% Plot results figure; plot(t, y(:,1), '-b', 'DisplayName', 'Prey'); hold on; plot(t, y(:,2), '-r', 'DisplayName', 'Predator'); xlabel('Time'); ylabel('Population'); title('Predator-Prey Dynamics'); legend show;
Step 3: Debugging and Testing
Common debugging strategies include:
-
Using the Command Window: Test small code snippets interactively.
-
Setting Breakpoints: Pause execution to inspect variables.
-
Checking Errors: Pay attention to MATLAB error messages.
For this project, validate the model by comparing the output against known results from literature or textbook examples. Need assistance with your matlab assignment help? Let Online give you the expert support you need to succeed.
Step 4: Enhancing Visualization
Improve visualization for better insights:
-
Use color-coding to distinguish between prey and predator populations.
-
Add gridlines and annotations to highlight key points.
-
Incorporate interactive elements, such as sliders for parameter adjustments.
Lessons Learned
Challenges Faced
This assignment presented several challenges:
-
Understanding the Mathematical Model: Translating equations into MATLAB syntax required careful attention.
-
Debugging Errors: Initial attempts produced unstable solutions due to incorrect parameter values.
-
Balancing Accuracy and Efficiency: Achieving precise results without excessive computation time was a delicate task.
Strategies for Success
Key takeaways from this experience include:
-
Plan Before Coding: Invest time in understanding the problem and designing an algorithm.
-
Incremental Development: Build and test your code in small sections.
-
Utilize MATLAB Resources: Refer to MATLAB’s documentation and community forums for guidance.
Conclusion
Tackling a complex MATLAB assignment can be a rewarding learning experience. By breaking the problem into manageable parts, leveraging MATLAB’s robust features, and applying systematic debugging, students can successfully overcome challenges and deliver high-quality results. This case study underscores the importance of a structured approach and persistence in mastering MATLAB assignments.
0 Comments
Post Comment